如何使用 Keywords 限制 WordPress / WooCommerce 用户注册
最近,我们的 WordPress 网站上搭建的 WooCommerce 店铺经常收到 Delivery incomplete
的邮件报错,点进去发现基本都如下的报错情况:
Delivery incomplete |
There was a temporary problem delivering your message to [email protected]. Gmail will retry for 46 more hours. You'll be notified if the delivery fails permanently. |
LEARN MORE |
意思就是邮件投递失败,查询后发现是机器人使用了随机的域名注册了店铺账户,收件箱内经常出现如下图的邮件提醒。
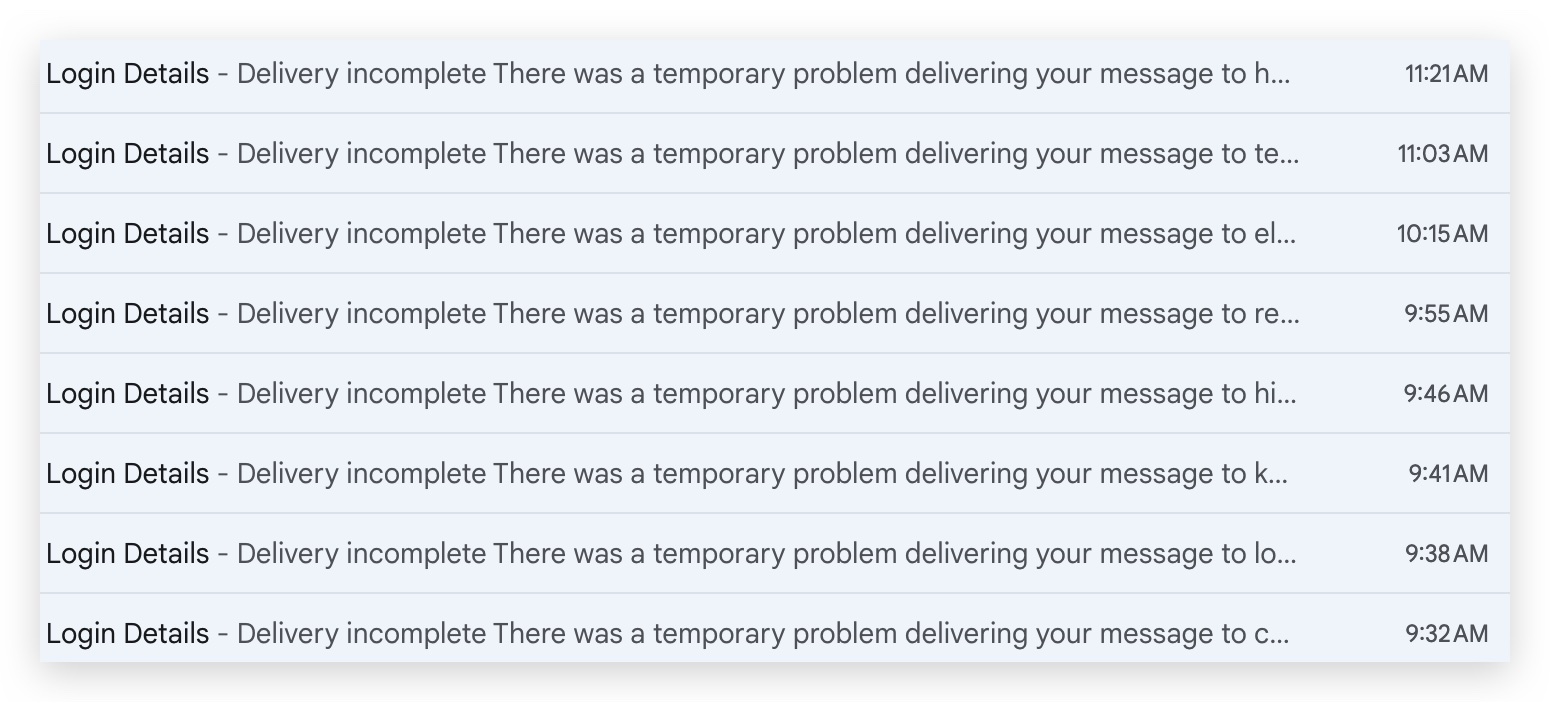
作为一个 商城网站,我们不能关停用户注册的功能,并且也使用了人机验证的服务,但是任然没法避免机器人注册泛滥,因此我们需要加上注册的限制功能。
我先简单搜索了下,确实有相关的插件可以解决此类问题,但是为了避免这类插件带来一些不必要的功能,因此我们采用自行添加代码到子主题的 functions.php
中实现相关的功能。
关键词设置界面
首先需要一个可以自定义关键词和限制规则的设置界面,设置的选项包括关键词设置和限制三级域名的邮箱注册。
// Register a new settings menu in WordPress admin
function register_limits_settings_menu() {
add_options_page( 'Registration Keywords Options', 'Registration Keywords', 'manage_options', 'registration-limits', 'registration_limits_settings_page' );
}
add_action( 'admin_menu', 'register_limits_settings_menu' );
// Define the layout and fields of the settings page
function registration_limits_settings_page() {
?>
<div>
<?php screen_icon(); ?>
<h2>Registration Keywords Settings</h2>
<form method="post" action="options.php">
<?php settings_fields( 'registration_limits_options' ); ?>
<?php do_settings_sections( 'registration_limits' ); ?>
<?php submit_button(); ?>
</form>
</div>
<?php
}
// Register the settings fields with WordPress
function register_keywords_settings_fields() {
add_settings_section( 'registration_limits_section', '', '', 'registration_limits' );
add_settings_field( 'restricted_keywords', 'Restricted Keywords', 'restricted_keywords_callback', 'registration_limits', 'registration_limits_section' );
add_settings_field( 'ignore_3level_domain', 'Ignore 3-Level Domain Names', 'ignore_3level_domain_callback', 'registration_limits', 'registration_limits_section' );
register_setting( 'registration_limits_options', 'restricted_keywords', 'sanitize_text_field' );
register_setting( 'registration_limits_options', 'ignore_3level_domain', 'absint' );
}
add_action( 'admin_init', 'register_keywords_settings_fields' );
// Define the callback to display the "Restricted Keywords" field on the settings page
function restricted_keywords_callback() {
$restricted_keywords = get_option( 'restricted_keywords', '' );
?>
<input type="text" id="restricted_keywords" name="restricted_keywords" value="<?php echo esc_attr( $restricted_keywords ); ?>" />
<p class="description">Enter a comma separated list of keywords to restrict during registration.</p>
<?php
}
// Define the callback to display the "Ignore 3-Level Domain Names" checkbox on the settings page
function ignore_3level_domain_callback() {
$ignore_3level_domain = get_option( 'ignore_3level_domain', 0 );
?>
<input type="checkbox" id="ignore_3level_domain" name="ignore_3level_domain" value="1" <?php checked( $ignore_3level_domain, 1 ); ?> />
<label for="ignore_3level_domain">Ignore registration if email address includes 3-level domain name.</label>
<?php
}
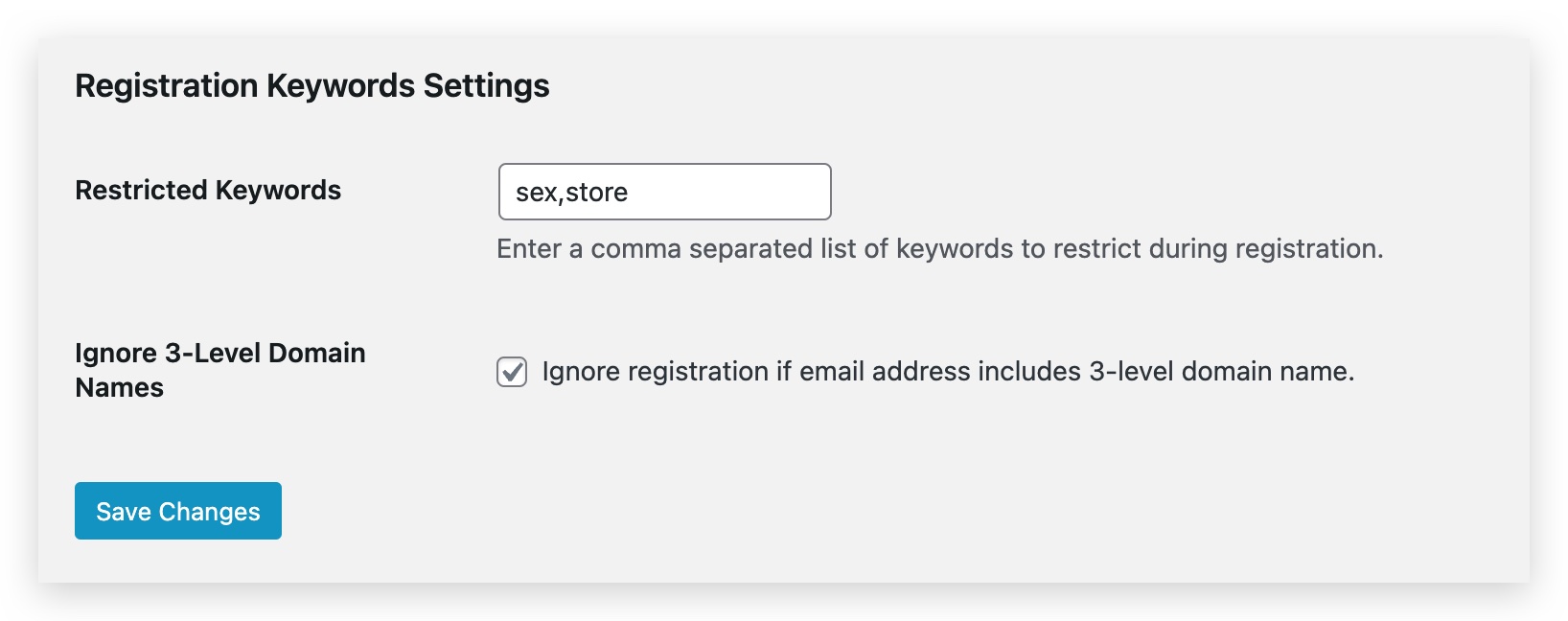
限制用户注册
只要满足以上2个条件的注册信息,都需要被屏蔽掉,我们需要采用 woocommerce_registration_errors
的 hook 进行限制。
// Validate the registration form and restrict based on keyword and 3-level domain name conditions
function restricted_keyword_in_email($errors, $sanitized_user_login, $user_email)
{
$ignore_3level_domain = get_option('ignore_3level_domain', 0);
// ignore 3-level domain name condition
if ($ignore_3level_domain == 1) {
$email_parts = explode("@", $user_email);
$domain_parts = explode(".", $email_parts[1]);
if (count($domain_parts) == 3) {
$errors->add('email_invalid', __('Invalid email address containing 3-level domain name.'));
}
}
// restrict keyword condition
$restricted_keywords = get_option('restricted_keywords', '');
$restricted_keywords_array = explode(',', $restricted_keywords);
foreach ($restricted_keywords_array as $restricted_keyword) {
$restricted_keyword = trim($restricted_keyword);
if (strpos($user_email, $restricted_keyword) !== false) { // check for presence of keyword
$errors->add('email_invalid', __('Invalid email address containing restricted keyword.')); // throw error
}
}
return $errors;
}
add_filter('woocommerce_registration_errors', 'restricted_keyword_in_email', 10, 3);
拦截效果演示
-
注册限制关键词
-
注册限制三级域名